This page details how to connect your application to a GameWisp channel.
Did you Register an Application?
Don't forget that you need to have an application registered to complete the next steps. Check out the Registering an Application section to see how.
Quick Start
To connecting to a GameWisp channel, your application will need to perform these five basic steps:
- Build a url to the authorize endpoint and put it in a link on a page on your site.
- Once the user clicks on that link, they’ll be directed to the /oauth/authorize endpoint on GameWisp.
- The user will either approve access to your app or decline. In either case, they’ll be redirected to the redirect_uri you specify with an authorization code (in the case of approval) or an error (in the case of denying access).
- If the user approved access, take the code parameter and use the /oauth/token endpoint to receive an access_ token and a refresh_token.
Keep your Refresh Tokens up to Date!
A refresh token expires in 70 days, be sure that you're properly refreshing your access tokens before a refresh token's expiry or you will be forced to re-authenticate users. This is required even if you're only using Singularity.
Walkthrough
Use a Comma to Split Multiple Scopes
If you require more than one scope, remember to use a comma as the scope delimiter. See the example code below.
Build a URL to the authorize endpoint located at /api/v1/oauth/authorize and put it somewhere that a user can click on it.
<?php
$query = http_build_query([
'response_type' => 'code',
'client_id' => 'YOUR_CLIENT_ID',
'clientSecret' => 'YOUR_APPLICATIONS_SECRET',
'redirect_uri' => 'YOUR_CLIENT_REDIRECT_URI',
'scope' => 'read_only',
'state' => 'base64 encoded state string -- required, can be meaningless'
]);
header("Location: https://api.gamewisp.com/pub/v1/oauth/authorize?$query");
exit;
//The following example uses NodeJS with Express and the simple-oauth2 packages.
var app = require('express')();
var oAuthInfo = {
site: 'https://api.gamewisp.com',
clientID: 'your client ID',
clientSecret: 'your client secret',
tokenPath: '/pub/v1/oauth/token',
authorizationPath: '/pub/v1/oauth/authorize',
};
var oauth2 = require('simple-oauth2')(oAuthInfo);
// Authorization uri definition
var authorization_uri = oauth2.authCode.authorizeURL({
redirect_uri: 'your-redirect-uri',
scope: 'read_only',
state: 'base64 encoded state data, passed back to you'
});
//redirects to the gamewisp authorize page.
app.get('/auth', function(req, res){
res.redirect(authorization_uri);
});
https://api.gamewisp.com/pub/v1/oauth/authorize?client_id=CLIENT_ID_HERE&redirect_uri=REDIRECT_URI_HERE&response_type=code&scope=read_only&state=ASKDLFJsisisks23k
$code = $_GET['code'];
$post = [
'grant_type' => 'authorization_code',
'client_id' => 'YOUR_CLIENT_ID',
'client_secret' => 'YOUR_CLIENT_SECRET',
'redirect_uri' => 'YOUR_REDIRECTION_HERE',
'code' => $code,
'state' => 'AXJDJSS'
];
$ch = curl_init('https://api.gamewisp.com/pub/v1/oauth/token');
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, $post);
$response = curl_exec($ch);
curl_close($ch);
$result = json_decode($response);
/** Now that we've parsed the returned data, here are your tokens **/
$access_token = $result->data->access_token;
$refresh_token = $result->data->refresh_token;
Once the user clicks the URL, they'll be taken to a page that looks like the following:
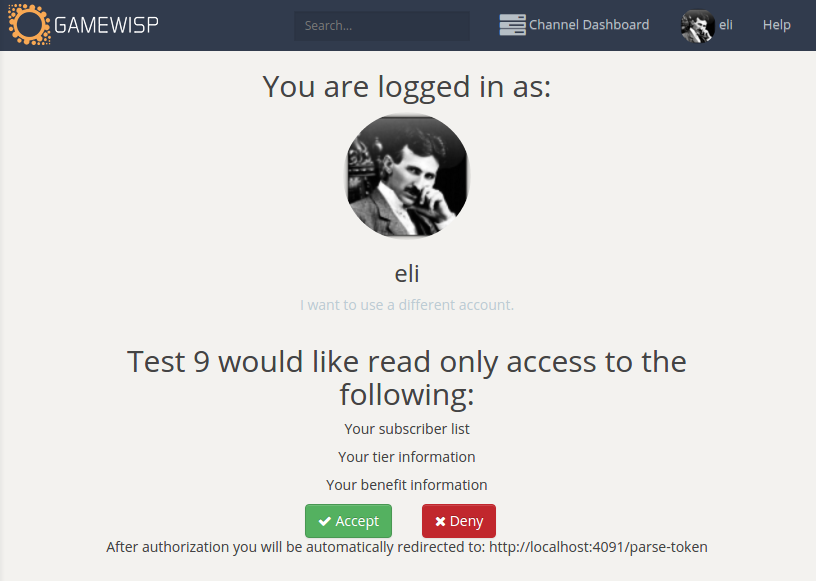
The authorization page displayed for the user after clicking an authorization url.
Regardless of whether or not the user clicks "Accept" or "Deny" they will be redirected back to the Redirect URL you supplied when you initially registered your application with GameWisp.
If the user clicks "Deny" they will be redirected and an error message will be passed to your Redirect URL. If the user clicks "Accept" they will be redirect and an authorization code and your state parameter will be passed back to your Redirect URL.
Once you receive the authorization code, send it to the /oauth/token endpoint to exchange the code for a token.
// assume $code is obtained via redirect from the user visiting a url of the following structure:
// https://gamewisp.com/pub/v1/oauth/authorize?client_id=CLIENT_ID_HERE&redirect_uri=REDIRECT_URI_HERE&response_type=code&scope=read_only
//if using Guzzle 6+ change "body" to "form_params"
$response = $guzzleClient->post('https://gamewisp.com/pub/v1/oauth/token', [
'form_params' => [
'grant_type' => 'authorization_code',
'client_id' => 'your client ID',
'client_secret' => 'your client secret',
'redirect_uri' => 'your redirect uri',
'code' => $code,
'state' => 'ADFASAWW'
]
]);
$result = json_decode($response->getBody());
//The following example uses NodeJS with Express and the simple-oauth2 packages.
var app = require('express')();
var oauth2 = require('simple-oauth2')(oAuthInfo);
app.get('your-redirect-uri', function(req, res){
var token = oauth2.authCode.getToken({
code: code,
redirect_uri: 'your-redirect-uri'
}).then(function saveToken(result){
token = oauth2.accessToken.create(result);
accessToken = token.token.data.access_token;
//store and use the token...
}).catch( function logError(error){
//your error catching here.
});
//token structure is as follows:
token:
{
result: { status: 1, message: 'Token issued' },
data:
{
access_token: 'the-access-token',
token_type: 'Bearer',
expires_in: 3600,
refresh_token: 'the-refresh-token'
},
expires_at: token-expiry-time (UTC)
}
After exchanging a code for a token, then token JSON object will contain an access_token and a refresh_token.
Working with Refresh Tokens
Refresh Tokens Expire
Refresh tokens expire after 70 days. Be sure to update the refresh token before the 70 day period or you will have to re-authenticate users manually.
You will need to use the refresh_token to update the access_token when it expires. Access tokens are updated by passing the refresh_token to the /oauth/token endpoint.
// if using Guzzle 6+ change "body" to "form_params"
$response = $guzzleClient->post('https://gamewisp.com/pub/v1/oauth/token', [
'body' => [
'grant_type' => 'refresh_token',
'client_id' => 'your client ID',
'client_secret' => 'your client secret',
'redirect_uri' => 'your redirect uri',
'refresh_token' => 'the refresh token'
]
]);
$result = $response->json();
//The following example uses NodeJS with the simple-oauth2 packages.
var oAuthInfo = {
site: 'https://gamewisp.com',
clientID: 'your client ID',
clientSecret: 'your client secret',
tokenPath: '/pub/v1/oauth/token',
authorizationPath: '/pub/v1/oauth/authorize',
};
var oauth2 = require('simple-oauth2')(oAuthInfo);
// Sample of a JSON access token you obtained through previous steps
var token = {
'access_token': 'access_token',
'refresh_token': 'refresh_token',
'expires_in': '3600'
};
var token = oauth2.accessToken.create(token);
if (token.expired()) {
token.refresh(function(error, result) {
token = result;
});
}
Upon proper exchange of the refresh token, you will receive a response of the following format
{
"result": {
"status": 1,
"message": "Token issued"
},
"data": {
"access_token": "new-access-token",
"token_type": "Bearer",
"expires_in": 3600,
"refresh_token": "new-refresh-token"
}
}
Store the new Refresh Token!
New refresh tokens are randomly generated when exchanging your current refresh token for a new access token. Be sure to store the new refresh_token too.
Be sure to update your access_tokens before your refresh tokens expire. The best way to do this is likely with some sort of nightly job that updates the access_tokens of connected users.
If a refresh_token expires before you use it, you will be forced to manually reauthenticate the connected user, typically by requiring the user to come back to your application and authorize it again.
What Next?
That's it! You've successfully authorized your client access to a GameWisp channel's data. Now check out the RESTful API and Singularity sections of this documentation to see what you can do.